Python you can be proud of
Contents
Python you can be proud of#
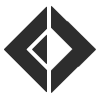
Immutable by default. Powerful pattern matching. Lightweight syntax. Units of measure. Type providers. Enjoy.
Type inference provides robustness and correctness, but without the cost of additional code. Let the compiler catch bugs for you.
Fable produces readable Python code compatible with PEP8 standards and also uses type-hints.
Call Python from Fable or Fable from Python. Use PyPI packages. The entire Python ecosystem is at your fingertips.
Choose your favorite tool: from Visual Studio Code to JetBrains Rider. Check the whole list here.
Fable supports the F# core library and some common .NET libraries to supplement the JavaScript ecosystem.
See also
Features#
These are some of the main F# features that you can use in your web apps with Fable.
type Face =
| Ace | King | Queen | Jack
| Number of int
type Color =
| Spades | Hearts | Diamonds | Clubs
type Card =
| Face * Color
let aceOfHearts = Ace,Hearts
let tenOfSpades = (Number 10), Spades
match card with
| Ace, Hearts ->
printfn "Ace Of Hearts!"
| _, Hearts ->
printfn "A lovely heart"
| (Number 10), Spades ->
printfn "10 of Spades"
| _, (Diamonds|Clubs) ->
printfn "Diamonds or clubs"
// Warning:
// Incomplete pattern matches on this expression.
// For example, the value '(_,Spades)' may indicate
// a case not covered by the pattern(s).
Computational expressions#
There’s a lot of code involving continuations out there, like asynchronous or undeterministic operations. Other languages bake specific solutions into the syntax, with F# you can use built-in computation expressions and also extend them yourself.
// Python async made easy
task {
let! res = Fetch.fetch url []
let! txt = res.text()
return txt.Length
}
// Declare your own computation expression
type OptionBuilder() =
member __.Bind(opt, binder) =
match opt with
| Some value -> binder value
| None -> None
member __.Return(value) =
Some value
let option = OptionBuilder()
option {
let! x = trySomething()
let! y = trySomethingElse()
let! z = andYetTrySomethingElse()
// Code will only hit this point if the three
// operations above return Some
return x + y + z
}
Units of measure#
These are some of the main F# features that you can use in your web apps with Fable.
[<Measure>] type m
[<Measure>] type s
let distance = 12.0<m>
let time = 6.0<s>
let thisWillFail = distance + time
// ERROR: The unit of measure 'm' does
// not match the unit of measure 's'
let thisWorks = distance / time
Type providers#
Build your types using real-world conditions and make the compiler warn you if those conditions change.
open Zanaptak.TypedCssClasses
type Bulma = CssClasses<"https://cdnjs.cloudflare.com/ajax/libs/bulma/0.9.3/css/bulma.min.css", Naming.PascalCase>
let hero (model: Model) body =
Html.section [
prop.classes [ Bulma.Hero; Bulma.IsFullheightWithNavbar ]
prop.style [
style.backgroundImageUrl (model.Banner)
style.backgroundPosition "center"
style.backgroundSize.cover
]
prop.children [
Html.div [
prop.classes [ Bulma.HeroBody; Bulma.IsDark ]
prop.children [ Html.div [ prop.classes [ Bulma.Container ]; prop.children body ] ]
]
]
]